Opensend
Integration
The following is a guide on how to integrate Opensend with Bloomreach Engagement using Omniconnect.
Opensend enables brands to identify anonymous website traffic by collecting the first name, surname, email address, physical postal address, and device data from its identity graph. This enables real-time retargeting and drives higher conversions from first-time and repeat visitors.
The service matches data for United States traffic by adding a pixel to your website. It connects to Bloomreach Engagement and sends retargeting messages to reclaim potential lost revenues while improving anonymous traffic match rates.
Prerequisites
- A live Bloomreach Engagement account and an Opensend trial account or live account.
- To integrate Opensend with Custom webhook, you will need:
- Webhook method,
- Authorization (if needed),
- Webhook URL.
How to set it up
The Opensend integration involves a two-step process:
- Install a pixel on your website,
- Integrate the delivery service using a custom webhook to send resolved contacts to Bloomreach.
Install Opensend Pixel on your website
To start tracking anonymous visitors from your website and get the Bloomreach Cookie for contacts from the website, paste the following code in the <head>
section of your website.
__exponea_etc__
will be added as a custom field on Opensend to pass to Bloomreach for Cookie Sync.
<script async>
!function(s) {
var o = s.createElement('script'), u = s.getElementsByTagName('script')[0];
o.src = 'https://cdn.aggle.net/oir/oir.min.js';
o.async = !0, o.setAttribute('oirtyp', 'XXXXXXXX'), o.setAttribute('oirid', 'XXXXXXXX');
u.parentNode.insertBefore(o, u);
}(document);
</script>
<script async>
!function(e) {
// Wait for __exponea_etc__
var checkInterval = setInterval(function() {
var exponeaEtc = document.cookie.split(";").map(c => c.trim()).filter(c => {return c.substring(0, 16) == '__exponea_etc__=';}).map(c => {return decodeURIComponent(c.substring(16));})[0] || null
if (exponeaEtc !== null) {
// Clear the interval, stop the loop
clearInterval(checkInterval);
// Set custom-fields and trigger Onsite event
var t = {
source: 'Opensend',
__exponea_etc__: exponeaEtc
};
(e._oirtrk = e._oirtrk || []).push(['track', 'on-site', t]);
} else {console.log(`__exponea_etc__: {$exponeaEtc}`)}
}, 500); // Repeat every 500 milliseconds
}(window);
</script>
Set up Omniconnect for Opensend data sync
Set up Omniconnect in Bloomreach Engagement
In your Bloomreach Engagement project:
- Go to Data & Assets > Integrations.
- Press + Add a new integration.
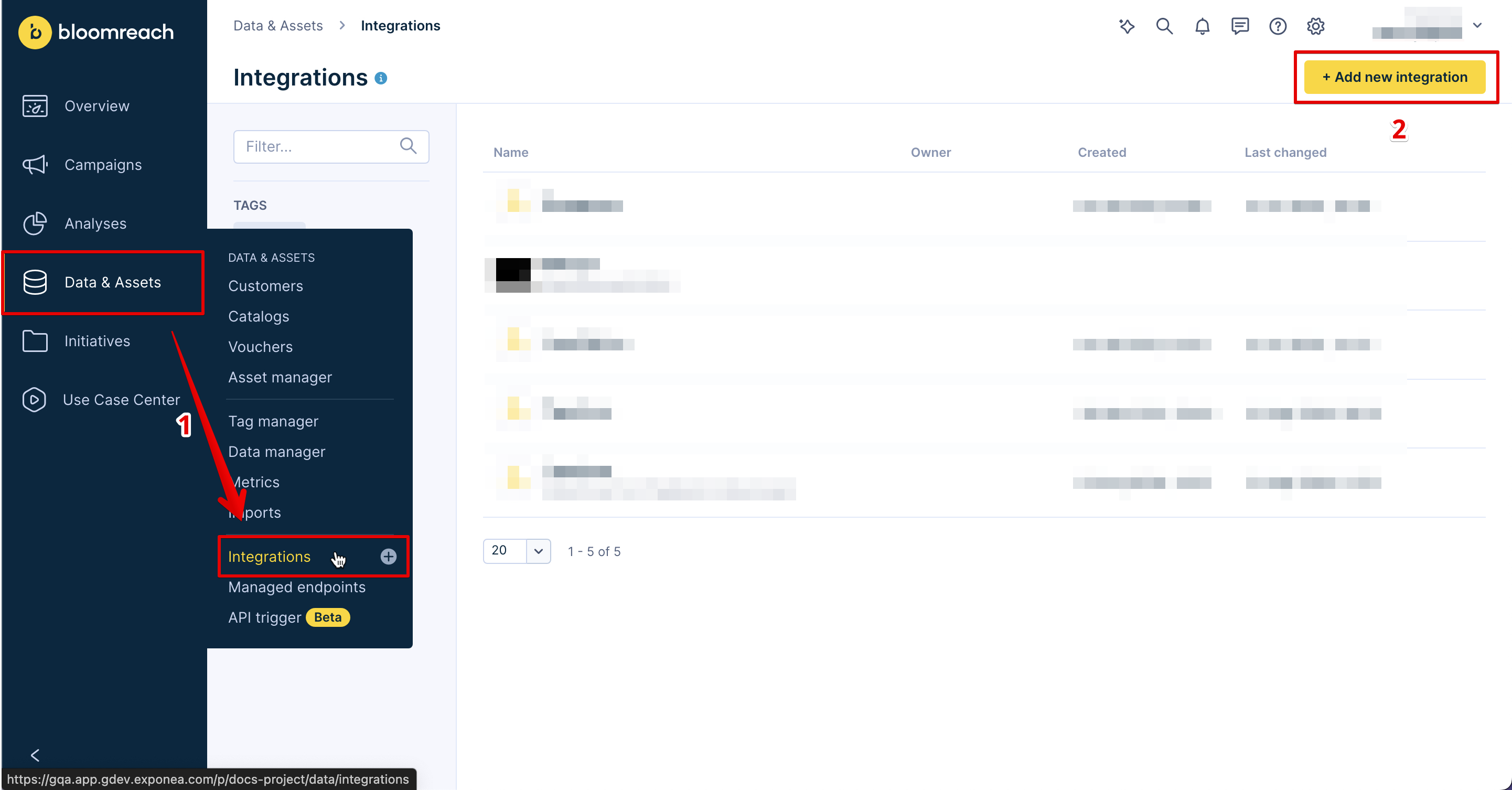
- Search for Omniconnect and press + Add integration.
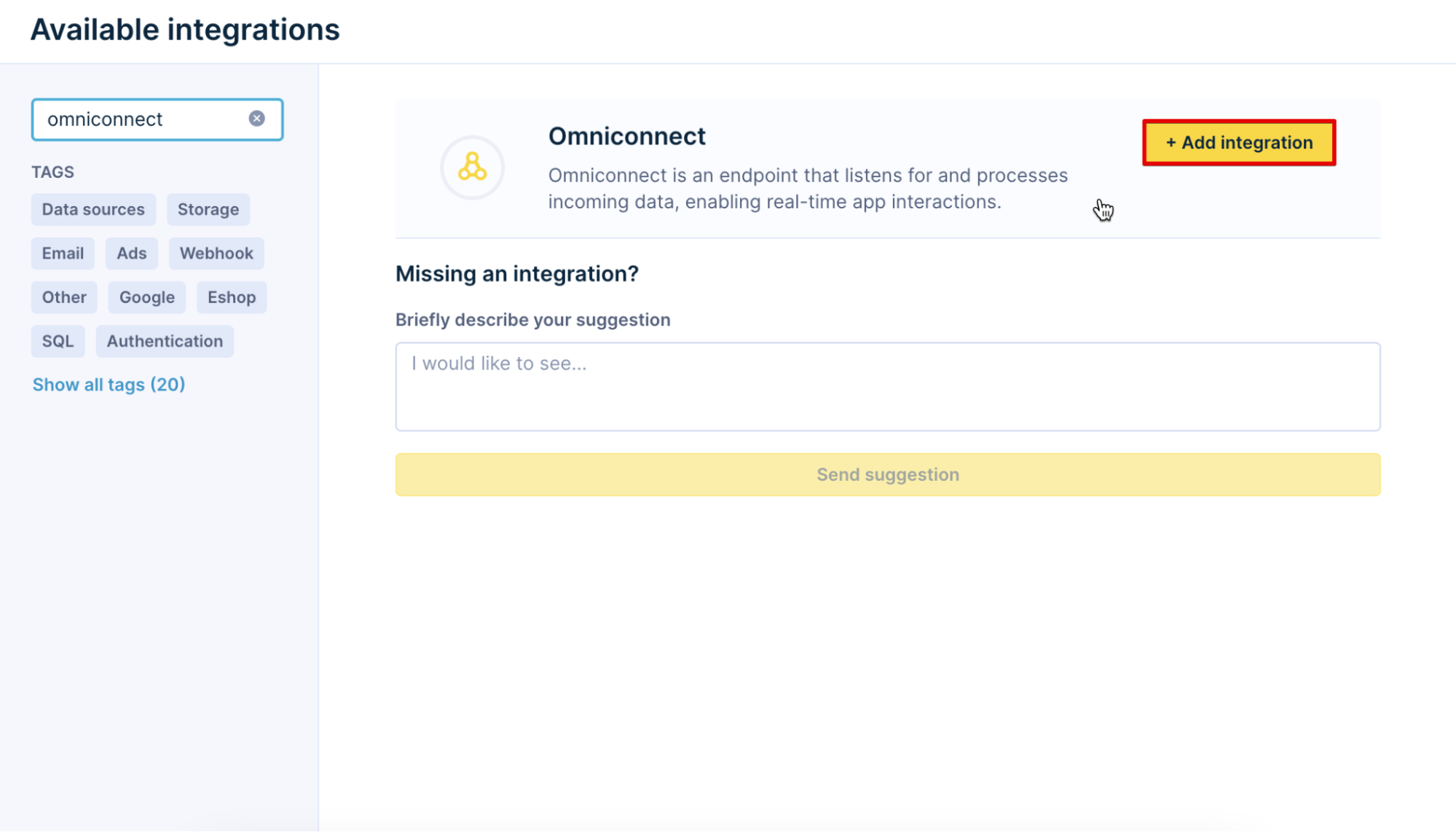
- Name the integration, for example, Opensend.
- Press Save integration.
- Provide a description that explains the function of this integration, for example, sync data from Opensend to Bloomreach Customer Profiles.
- Copy and save the Omniconnect URL. You will need it later.
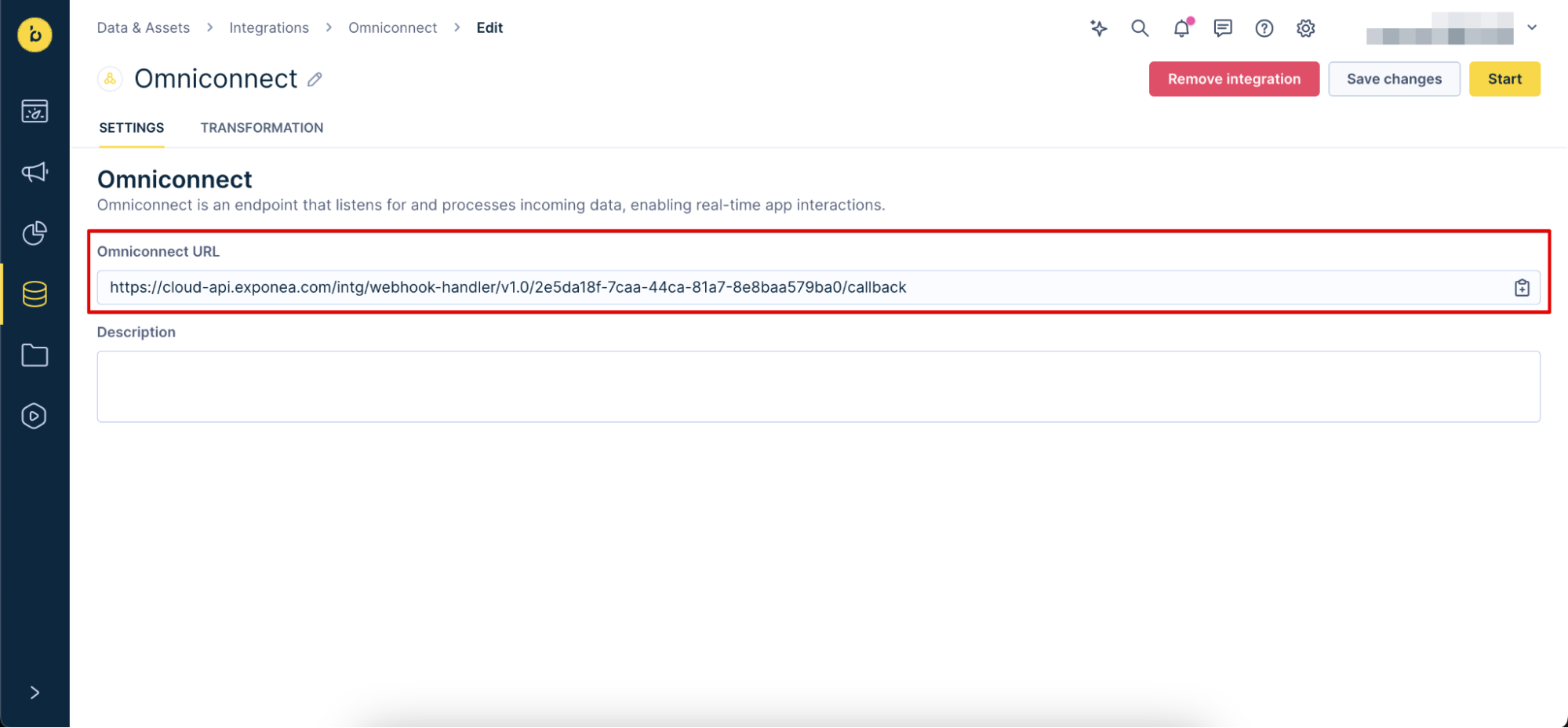
Set up a custom webhook in Opensend
To integrate Opensend to your Bloomreach account using custom webhook integration:
- Go to your Opensend Dashboard.
- Go to the Integrations > Main connections.
- Press + Add destination.

- Select Custom webhook.
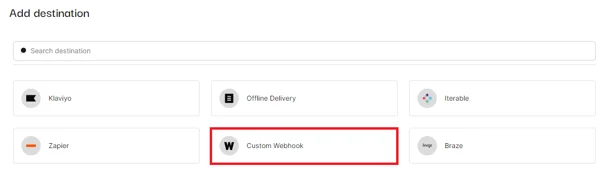
- Add a Name and Description.
- Press Next.
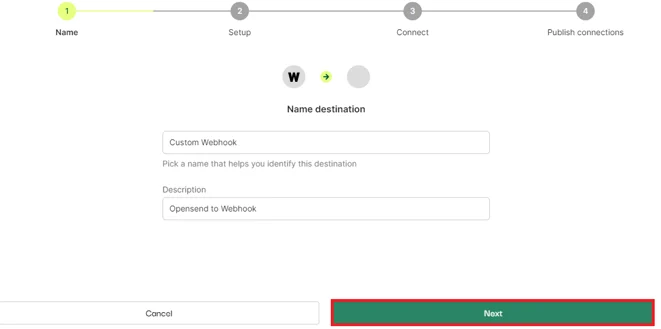
- Select the Custom webhook Webhook configuration.
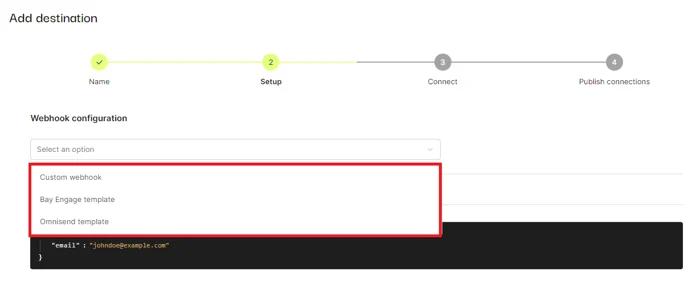
- Select the POST Webhook method.
- Select your Authorization method and fill out necessary details:
- API Key
- Oauth2
- From the Fields dropdown, select any default fields from Opensend and any custom fields you want to send to the Webhook.
- Add the Custom Webhook URL (Omniconnect URL from before) and press Send a test request.
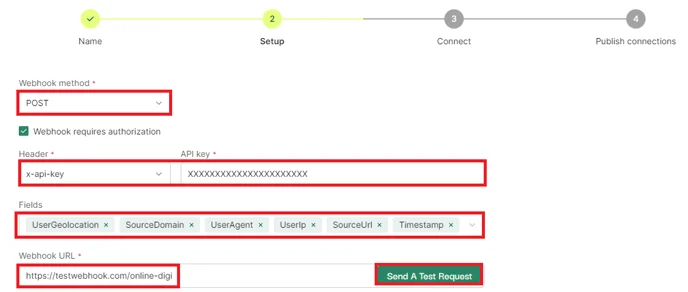
- In the popup, add the test email address to send the Webhook to and press Send.
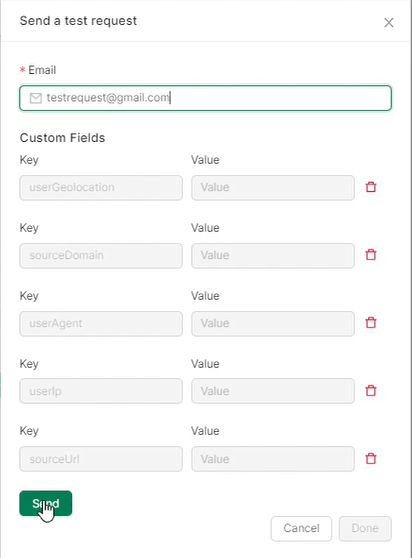
- The Headers section contains the test request sent to your Webhook URL with the test email, custom fields, and authorization details.
- With the test request, you can see what data Opensend data is sending and map the data accordingly on your end.
- Press Done to close the popup.
- Press Next.
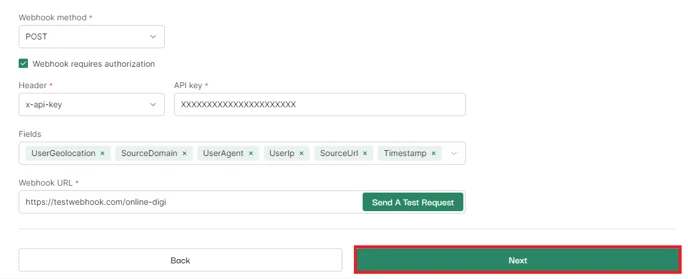
- Select the sources from where you want to receive the data.
- Press Create Connections.
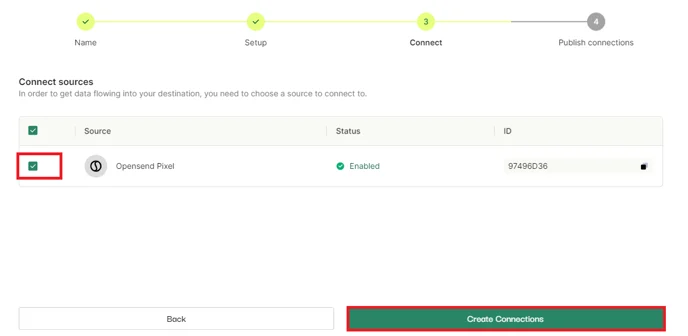
- Select the connections and press Publish.
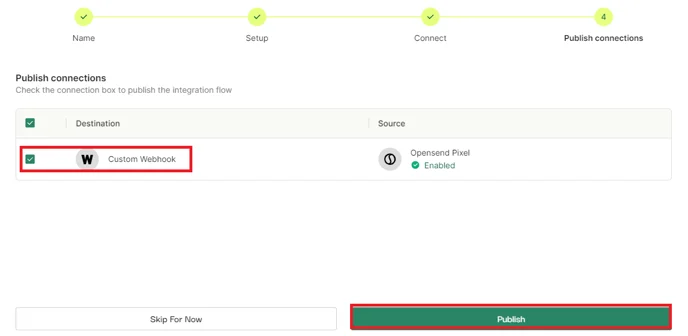
- Check the Example Request Data format.
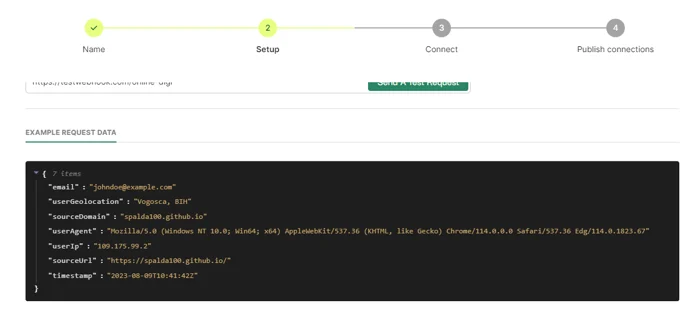
Transformation function
The next step is to write the transformation function. The transformation function allows for a high-level personalization of the integration using JavaScript.
Go to the Transformation tab in your Omniconnect integration in Bloomreach Engagement, paste the following function, and press Save:
/**
* The following code is a sample that can be used as a starter function using Omniconnect, except for the function name and the parameter you can modify the logic with the one that suits you the best.
*
* Things to consider before start:
* - We require the use of `const` and `let` instead of `var` to initialize variables
* - 3rd party libraries are not supported (on-demand)
* - console.log() is disallowed
*
* ***************************************************************
* Predefined Constants
*
* INTEGRATION_ID: As the name implies it's the identifier for the given webhook handler.
* COMPANY_ID: Project ID in Engagement Platform
* INTEGRATION_NAME: Name assigned to the current Webhook Handler integration.
*
* **********************************************************************
* Events
* The following is an example of the objects the `output` array can contain:
*
* CUSTOMER UPDATE
*
* {
* "name": "customers",
* "command_id": "abc",
* "data": {
* "customer_ids": {
* "registered": "[email protected]"
* },
* "properties": {
* "first_name": "Marian",
* },
* "update_timestamp": 1614941503 // Unix timestamp in seconds
* }
* }
* CUSTOMER EVENTS
* {
* "name": "customers/events",
* "command_id": "abc",
* "data": {
* "customer_ids": {
* "registered": "[email protected]"
* },
* "event_type": "purchase",
* "timestamp": "1614941503", // Unix timestamp in seconds
* "properties": {
* "total_price": 1234.50,
* }
* }
* }
*
* Do you want to know more? please visit the following link:
* - How the output is being processed https://documentation.bloomreach.com/engagement/reference/batch-commands-2
* - Why customer_ids are important? - https://documentation.bloomreach.com/engagement/docs/external-id
* - https://documentation.bloomreach.com/engagement/reference/add-event-2
*
* Output:
* It should be an array of object(s)
* [
* {}
* ]
*
*
*
* Happy coding :)
*/
// HINT: We can set constants in this way
const INTEGRATION_TYPE = "Opensend";
/**
* Handler is the entry point for the function that enables the transformation of the data sent to our platform.
* The input field should be a JSON structure `{}` and it's passed using the param `payload`. The name of the parameter can be changed but the number of parameters cannot be modified and should remain as in the current example.
*
* @param payload {JSON} JSON structure fetched from the `Input` field
* @returns Array.<Object> [{}] Array of events
*/
function handler(payload) {
const eventList = [];
/**
* External IDs (in this example customerIDs) are an important part of the event
* These IDs allow us to link the events with the right customers
* Read more about external ids: https://documentation.bloomreach.com/engagement/docs/external-id
**/
const customerIDs = {
"cookie": payload.__exponea_etc__,
"email_id": payload.email
};
/**************************************************************/
/* Customer Update */
/**************************************************************/
// Generate the properties we want to attach to the event
const transformedUpdateProps = {
"source":"Opensend",
"description": "Customer update should contain the following fields: name, command_id, data)",
"description_2": "'data' field should contain: customer_ids, properties, update_timestamp (UNIX timestamp)",
"Opensend_Source_URL": payload.sourceUrl,
"Opensend_GeoLocation": payload.userGeolocation,
"created_at": parseDateToTimestampInSeconds(payload.timestamp),
};
// Customer Update event contains all the fields required to publish a customer update
const customerUpdate = {
name: "customers", // see more: https://documentation.bloomreach.com/engagement/reference/add-event-2
command_id: `${payload.event_id || payload.email}-${payload.event_type || ''}`,
data: {
customer_ids: customerIDs, // HINT: Mandatory field
properties: transformedUpdateProps, // HINT: Mandatory field
update_timestamp: currentTimestampInSeconds(), // HINT: Mandatory field
},
};
eventList.push(customerUpdate);
/**************************************************************/
/* Customer Event */
/**************************************************************/
// Generate the properties we want to attach to the event
//const transformedEventProps = {
// "action": payload.customer_event_data.event_type,
// "integration_name": "integration",
// "description": "Customer event should contain the following fields: name, command_id, data",
// "description_2": "'data' field should contain the following fields: event_type, customer_ids (at least one), properties, timestamp (UNIX timestamp)",
// "link_to_the_documentation": "https://example.com/",
// "integration_id": INTEGRATION_ID, // HINT: INTEGRATION_ID is a built-in constant that can be used across the function
// "integration_type": INTEGRATION_TYPE, // Initialized above
// "survey_name": payload.customer_event_data.title,
// "survey_id": payload.customer_event_data.form_id,
// "submitted_at": parseDateToTimestampInSeconds(payload.customer_event_data.submitted_at),
//};
// If tags values contain a value in the first position add it in the `first_tag` property
//if (payload.customer_event_data.tags[0]) {
// transformedEventProps["first_tag"] = payload.customer_event_data.tags[0];
//}
// Customer Event contains all the fields required to publish a customer event
//const customerEvent = {
// name: "customers/events",
// data: {
// event_type: payload.customer_event_data.event_type, // HINT: Mandatory field
// customer_ids: customerIDs, // HINT: Mandatory field
// properties: transformedEventProps, // HINT: Mandatory field
// timestamp: currentTimestampInSeconds(), // HINT: Mandatory field
// },
//};
//eventList.push(customerEvent);
// eventList should return an array of event [{}]
return eventList;
}
/**
* Function that helps us generate the timestamp in the format that is required by Engagement
*
* The timestamp can be updated to fit with your needs, the current example is generating the time from the time when the function is executed - Date.now()
* e.g.
* Function executed on Mon Jan 01 2024 11:00:00 GMT+0000 -> returns -> 1704106800
*
* @returns number Unix timestamp
*/
function currentTimestampInSeconds() {
return Math.round(Date.now() / 1000);
}
/**
* Function that helps us generate the timestamp in the format that is required by Engagement from a timestamp passed in the format 2024-01-11T11:11:11Z from the time when the function is executed - Date.now()
* This function is just for demo purposes of how we can parse date and normalize it to the UNIX Timestamp format
* e.g.
* Param in the format 2024-01-11T11:11:11Z -> returns -> 1704106800
*
* @returns number Unix timestamp
*/
function parseDateToTimestampInSeconds(dateStr) {
const date = new Date(dateStr);
return Math.round(date.getTime() / 1000);
}
Track the integration
Once you are happy with the transformation part:
- Press Start to start your integration.
- Go to Data & Assets > Customers to track the events coming to Bloomreach from the platform. The data from the platform will automatically update customer profiles.
(Optional) Turn on notifications
Whenever the integration encounters an issue, you will be notified via email and in-app notification. We recommend you turn on the notifications in case your integration encounters any errors.
Note
Make sure your project's email notification settings are enabled. To turn them on, go to User settings > Notification settings and check the Get notifications via email box.
- Go to your Omniconnect integration.
- Press the three dots next to the Start button.
- Press Notifications.
- Enter the email addresses of relevant project users you wish to subscribe to Omniconnect notifications. We recommend adding your implementation partner, internal teams, or technical teams.
- Press Save.
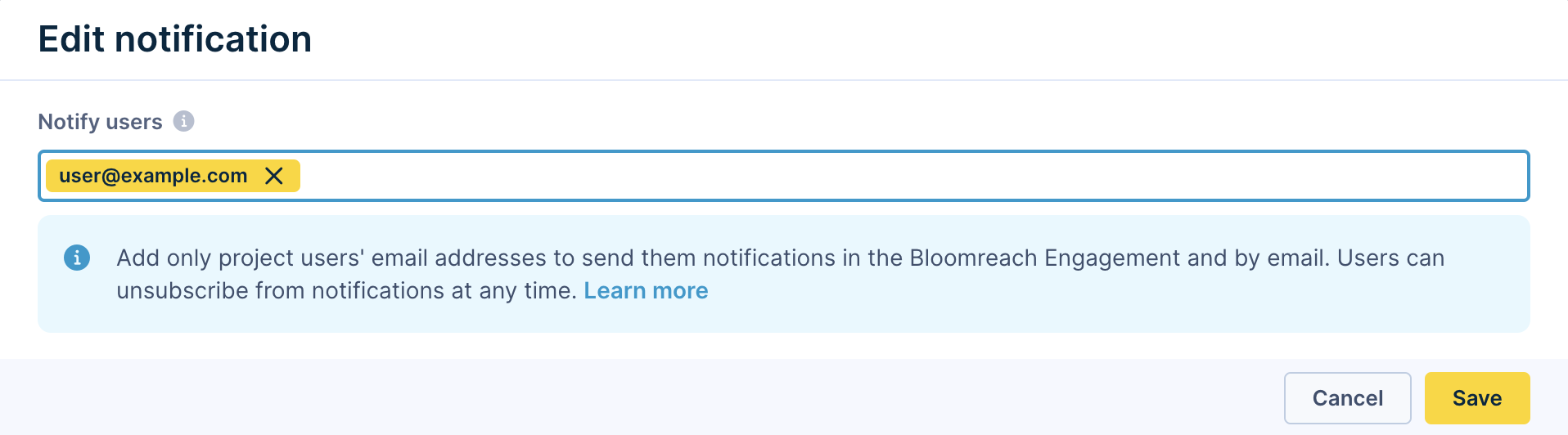
Updated 5 months ago