Integration of Recommendations
Requesting Recommendations
In order to get recommendations, you need to use the suggested getRecommendation()
method from the JavaScript SDK library or directly use our REST API.
You can find the code for requesting product recommendations using the Javascript SDK in the Web Deployment part of each of the recommendation engines.
Two versions of the code are available. One with preparation for an AB test, one without it.
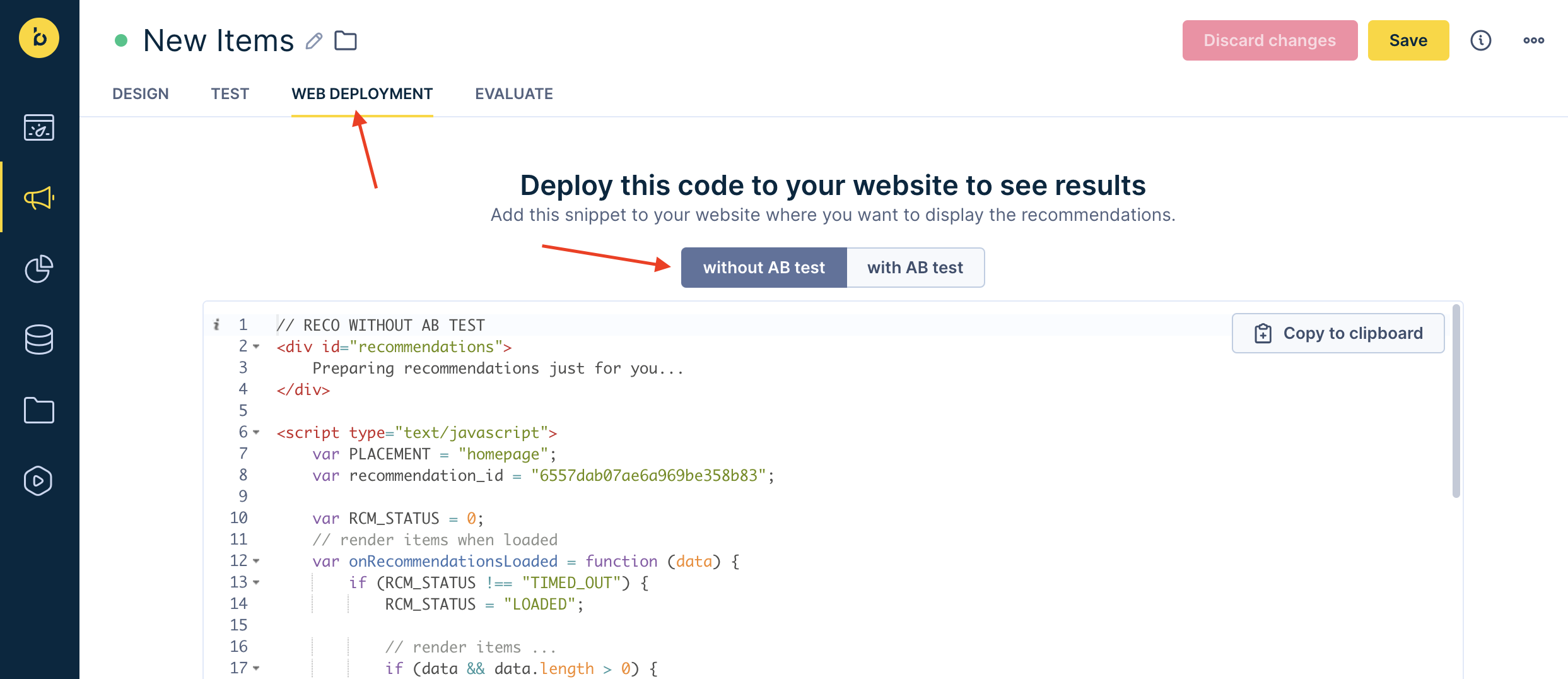
The full specification of the request can be found in the personalization guide, but below we describe the most important parts attributes:
Key | Value | Description | Example |
---|---|---|---|
recommendationId (Required) | String | Engine ID of an existing recommendation model. This ID is created when the engine is saved in Bloomreach Engagement. | '5a7c4dfefb6009323d4c7311' |
fillWithRandom, also only in Jinja:fill_with_random | Boolean | If true and there are not enough recommended items by the model, Bloomreach Engagement fills the gap with random items from the catalog until the required size is reached. (NB. can also be fillWithRandom) | 'true' |
size | Integer (Default value: 10) | Number of recommended items to return. The maximum suggested size to use is 100. | '50' |
items | Object | Information about currently viewed product by the browsing user. Represented as dictionary 'product_id: weight.' | {'item_123': 1} |
catalogFilter, also only in Jinja: catalog_filter | Array of Objects | Dynamically adds additional constraints to the template's catalog filter (first step when setting the recommendations engine) when retrieving recommended items. The filter applies to the list returned by the recommendation. | [ { "property": "category_2", "constraint": { "type": "string", "operator": "contains", "operands": [{ "type": "constant", "value": "shoes" }] } } ] |
categoryNames (Required for Metric based category) | Array (Max size: 10) | Currently viewed category or categories by the browsing user. | ['shoes', 'high heels'] |
The recommendationid in the recommendation call must be a constant string when recommendations are called in Jinja (in emails, blocks, weblayers, managed endpoints and other assets).
Variables or parameters cannot be used instead of the recommendationid.
When recommendations are retrieved via Javascript SDK or backend API, there, of course, is not such limitation.
Integration to campaigns
The following two Jinja code snippets demonstrate how to integrate recommendation engines into campaigns. One example shows a simple and another one advanced usage with an example engine ID 5a7c4dfefb6009323d4c7311
. Properties of retrieved items can be later obtained by calling {{ item.<attribute> }}
convention.
<ul id="recommendations">
{% for item in recommendations('5a7c4dfefb6009323d4c7311') %}
<li>
<a href="{{ item.url }}">{{ item.title }}</a>
</li>
{% endfor %}
</ul>
The above snippet processes obtained recommendations (=10 items) from the engine 5a7c4dfefb6009323d4c7311 and integrates them into HTML.
<ul id="recommendations">
{% for item in recommendations('5a7c4dfefb6009323d4c7311',
fill_with_random = true,
size = 50,
items = {"item_123": 1},
category_names = ["shoes", "men"], # use only for Category type of models
catalog_filter = [{
"property": "category_2",
"constraint": {
"type": "string",
"operator": "contains",
"operands": [{
"type": "constant",
"value": "shoes"
}]
}
}]
) %}
<li>
<a href="{{ item.url }}">{{ item.title }}</a>
</li>
{% endfor %}
</ul>
This snippet calls 50 products including a context item item_123 in the request that is already filtered down by the category_2 contains "shoes"
rule.
If the recommendation model cannot return enough products, fill_with_random = true
will fill the gap with randomly selected products that match the catalog filter conditions.
Types and operators for catalog_filter
catalog_filter
Type = "string"
Operators:
- is set
- is not set
- has value
- has no value
- equals
- does not equal
- in
- not in
- contains
- does not contain
- starts with
- ends with
- regex
Example for type "string"
catalog_filter = [{
"property": "category_2”,
"constraint": {
"type": "string",
"operator": "in",
"operands":
[{'type': 'constant', 'value': 'ABC'},
{'type': 'constant', 'value': 'DEF'},
{'type': 'constant', 'value': 'GHI'},
{'type': 'constant', 'value': 'JKL'}]
}
}]
Type = "number"
Operators:
- equal to
- in between
- less than
- greater than
- is set
- is not set
- has value
- has no value
Example for type "number"
catalog_filter = [{
"property": "price",
"constraint": {
"type": "number",
"operator": "in between",
"operands":
[{"type": "constant", "value": "1"}
{"type": "constant", "value": "200" }]
}
}]
For more information, see Personalization in Jinja section.
Limitations
Catalog filter cannot be applied to Filter-based recommendation model.
How to evaluate Product recommendations
It is suggested to compute metrics revenue per visitor and click through rate using Reports. A good practice is to carry out an AB test, either with your current model or if you do not have any, with any other model from Bloomreach Engagement. To learn how to set up the report, see our guide to AB Test Basic Evaluation. In order to track recommendation-attributed revenue, please implement event tracking for clicks on any product which was generated by Engagement product recommendations.
Event tracking for Recommendations performance
To evaluate the effectiveness of product recommendations, it is necessary to implement event tracking for product purchases, product views, and clicks on recommended products. The following snippet demonstrates how to track these events:
- A/B test: Conduct an A/B test to compare the performance of different recommendation models.
- Load recommendations using a timeout: : Load recommendations and set a timeout to handle cases where recommendations are not loaded in time.
- Track events
- Track the
recommendation
event with action=show when recommended items are loaded. - Track the
recommendation
event with action=timeout when loading times out (1000ms). - Track the
recommendation
event with action=click when an item is clicked (track every time, on both recommended and default items).
Remember to insert your recommendation_id
in the code. See how to find the ID.
Note
"Clicks" aren't natively tracked for email campaigns or other use cases with recommendations. Custom code is required to track these events. Ensure that you implement the necessary tracking code to capture click events in these scenarios.
How it works
- Go to the “Web deployment” section and use the script example or auto-generated snippet.
- Fetch the auto-generated snippet via the app. Then, you can make necessary adjustments with JS/HTML/CSS, such as:
- Removing unnecessary code
- Adjusting the events tracking according to their needs
- Adjusting A/B test variants.
Here is an example code snippet for integrating recommendations and tracking events:
var PLACEMENT = "homepage";
// get variant for AB test
exponea.getAbTest("rcm_" + PLACEMENT, {
"Variant A": 50, // Recommendations from Bloomreach Engagement
"Control Group": 50 // Default baseline
}, function (variant) {
if (variant == "Variant A") {
var RCM_STATUS = 0;
// render items when loaded
var onRecommendationsLoaded = function (data) {
if (RCM_STATUS !== "TIMED_OUT") {
RCM_STATUS = "LOADED";
// track event recommendation action=show
exponea.track("recommendation", {
action: "show",
variant: variant,
item_ids: itemIds,
// other properties: placement, recommendation_id, ...
});
// render items ...
// add click listeners ...
// track event recommendation action=click when item is clicked
exponea.track("recommendation", {
action: "click",
variant: variant,
item_id: itemId,
// other properties: placement, recommendation_id, ...
});
}
};
// start loading recommendations
var options = {
recommendationId: "RECOMMENDATION ID HERE",
callback: onRecommendationsLoaded,
// ... add optional parameters as required by your use case, i.e. item_id, size
// categoryNames in case of Metric based category engine
};
exponea.getRecommendation(options);
// start timeout to discard rendering when recommendations are loaded too late
setTimeout(function () {
if (RCM_STATUS !== "LOADED") {
RCM_STATUS = "TIMED_OUT";
// track event recommendation action=timeout
exponea.track("recommendation", {
action: "timeout",
variant: variant,
// other required properties: placement, recommendation_id, ...
});
}
}, 1000);
} else if (variant == "Control Group") {
// track event recommendation action=show
exponea.track("recommendation", {
action: "show",
variant: variant,
// other properties: placement, recommendation_id, ...
});
// add click listeners to fallback items ...
// track event recommendation action=click when item is clicked
exponea.track("recommendation", {
action: "click",
variant: variant,
item_id: itemId,
// other properties: placement, recommendation_id, ...
});
}
});
Using Recommendations in Campaigns
Recommendations are reused by default at all places within one campaign (email, SMS, MMS) to the same customer.
However, if you wish to display different recommendations within one campaign, you must set a parameter
cached=False
within the recommendation call, using this simple example:
<ul id="recommendations">
{% for item in recommendations('5a7c4dfefb6009323d4c7311') (cached=False) %}
<li>
<a href="{{ item.url }}">{{ item.title }}</a>
</li>
{% endfor %}
</ul>
Recommendations via Experiments
If you are interested in deploying recommendations without any coding via a predefined ready-to-use block, read our article about Web Recommendations Blocks which will walk you through the setup step-by-step.
Updated 13 days ago