In this section, we will walk you through a specific example that examines the process of making an API call step by step. In each step, we will go over all the necessary details.
Example definition
Goal: Track a consent change by a client from the backend. The client no longer wishes to receive SMS notifications and withdraws the respective consent.
Prerequisites:
- You have already defined a consent category for SMS marketing within the Bloomreach Engagement app Consent Management
- You are using registered as an ID to recognize the client
Example guide
Overview
Making a call consists of several steps. First, you will need to create a relevant API group and set up permissions. Then, you will need to create the method’s URL. Once that is done, you will need to build the authorization header and the body of the API call.
Step 1: Create a new API Group and set up Permissions
In order to work with consents, you will need to set up a new group with a private access type. To do so, follow the steps outlined in the Prerequisites section. It is advised that you name the group with your use case in mind, for example, "Consent change". After creating the group, do not forget to save the Secret key at a secure location, as you will only have a chance to view it once.
Now you will need to configure the permissions needed for working with consents. In the Events tab, tick both Get and Set. These should be all the permissions that you need for this use case. Following the access minimization principle, you should not allow more permissions than what is needed for the use case that you are using the API Group for.
Step 2: Put together a URL for the API call
To build the URL for the API call, you will need 3 things: the URL of the HTTP method (Add consent in this case), your base URL, and your project token.
First, navigate to our online API Reference and find the particular method. In this example, that would be Add consent, which is just a specific case of the Add event. At the top of the screen, you will find the URL that you need.
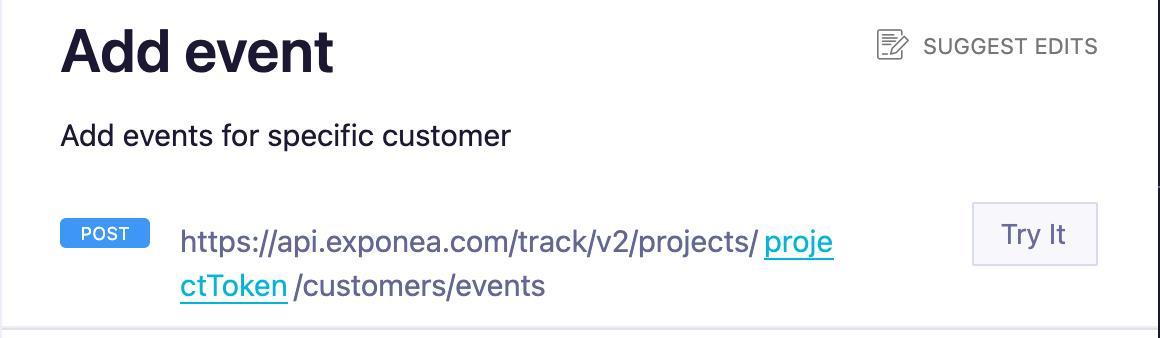
As you can see on the picture, for Add event, the URL is:
[base URL]/track/v2/projects/[projectToken]/customers/events
Notice that it has two parameters that you need to fill in: the base URL at the start and the project token in the middle. Both of these can be found and copied from Project Settings
> Access management
> API
. This is the same place where you have created your API group.
Now you have all the necessary components to build the URL for the API call. Simply exchange the parameters in the method’s URL for the respective values (base URL and project token). The final URL for changing the customer’s consent could look like this:
https://myinstance.exponea.com/track/v2/projects/12345678-abcd-1a2b-3c4d-123456789abc/customers/events
Step 3: Create an authorization header
Once you have the URL for the call, you will need to build the call’s header and body.
Because you are using the private access type, you will need to create a header using the Basic authentication that encodes the API Key ID / API Secret pair that Bloomreach Engagement has generated after creating the API group.
To do so, follow the steps outlined in the Authorization header section. Depending on the programming environment that you are using, the encoding process could vary. The following would be an example in PHP:
$auth_header = "Basic " . base64_encode($keyID . ":" . $secret)
Step 4: Build the body of the API call
To build the body of the API call, navigate back to our API Reference and read through our detailed description of the Add event request parameters and payload example. Then, based on the documentation, construct the body of the API call and fill out the parameters with the necessary information that you want to send in the call. You will need to add some specific fields for the event to be accepted as consent. You can find these in the Consent Management section in our documentation.
Example of an Add consent body
{
"customer_ids": { "registered": [customer_identificator] },
"event_type": "consent",
"timestamp": [time_of_the_consent_change],
"properties": {
"action": "reject",
"category": "sms_marketing"
}
}
Step 5: Send the API call!
You are now ready to send your first API call! You have configured all the permissions and prepared all the necessary components, including the URL, header, and body of the call. Now, you will have to put these together in the final request code and send the call. Note that in the production code, you will want to handle response codes and errors.
PHP example
<?php
require_once 'HTTP/Request2.php';
$request = new HTTP_Request2();
$request->setUrl('https://myinstance.exponea.com/track/v2/projects/12345678-abcd-1a2b-3c4d-123456789abc/customers/events');
$request->setMethod(HTTP_Request2::METHOD_POST);
$request->setHeader(array(
'content-type' => 'application/json',
'Authorization' => 'Basic MDEyMzQ1Njc4OWFiY2RlZmdoaWprbG1ub3BxcnN0dXZ3eHl6MDEyMzQ1Njc4OWFiY2RlZmdoaWprbG1ub3BxcjphYmNkZWZnaGlqa2xtbm9wcXJzdHV2d3h5ejAxMjM0NTY3ODlhYmNkZWZnaGlqa2xtbm9wcXJzdHV2d3h5ejAx'
));
$request->setBody('{"customer_ids": { "registered": "[email protected]" }, "event_type": "consent", "timestamp": 1620139769, "properties": {"action": "reject", "category": "sms_marketing"}}');
try {
$response = $request->send();
// Todo: handle response (success and error)
}
catch(HTTP_Request2_Exception $e) {
// Todo: handle exception
}